背景
新的文档采用Git+Markdown实现,本地需要一个好Markdown编辑器,最后选择使用vscode轻量级文本编辑器。
Markdown是一种轻量级的标记语言,虽然优化很多,但有两个明显的缺点:
- 图片添加及管理
- 表格添加及管理
表格可以采用在线文档替代,再分享或截图添加,图片的管理方式主要是有如下几种:
- 图片先上传到图床等cdn服务器,通过生成的网络地址引用
- 放置统一的目录,如根目录的img文件夹,再通过相对路径添加
- 通过文档名称创建文件夹,把markdown文件和图片资源统一放入
每种方式都各有优缺点,最终考虑到文档的可读性及图片管理,采用了方案2和3的融合方式:
1 2 3 4 5
| ├── 文档.md ├── img └── 文档 ├── a.png └── b.png
|
可以通过vscode的插件Paste Image实现从剪贴板自动复制图片到上述目录,如此插件有两个问题:
- 文件目录需要手动创建,不能自动创建
- 显示的图片不能修改显示大小,需要手动改为img标签来修改
通过git来管理文档还有比较严重的问题:无法快速的分享文档链接,需要通过web版git获取文档地址
需求及效果
需求如下:
- 从剪贴板自动复制图片,同时满足如下要求:
- 图片保存到以当前Markdown文件命名的文件里
- 通过img标签添加,默认width=500
- 获取当前文件的相对路径,并生成gitlab的在线地址
效果如下:
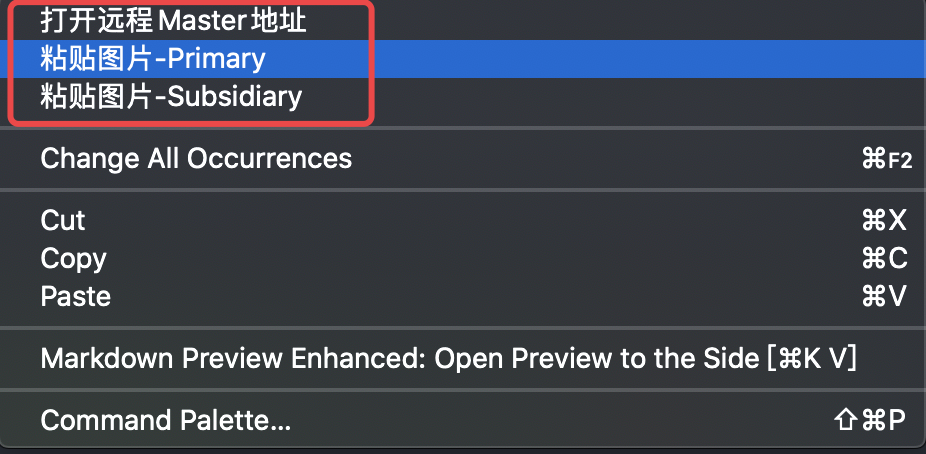
VSCode插件开发流程
参考官方文档:
- Your First Extension
- Publishing Extensions
阅读上面官方文档,通过代码脚手架,生成Hello World模板工程。
关键点:extension要选择typescript类型,方便复用第三方插件源码。
常用命令:
- F5:运行
- npm run publish:发布部署
vscode的插件开发很简单,如下图所示:
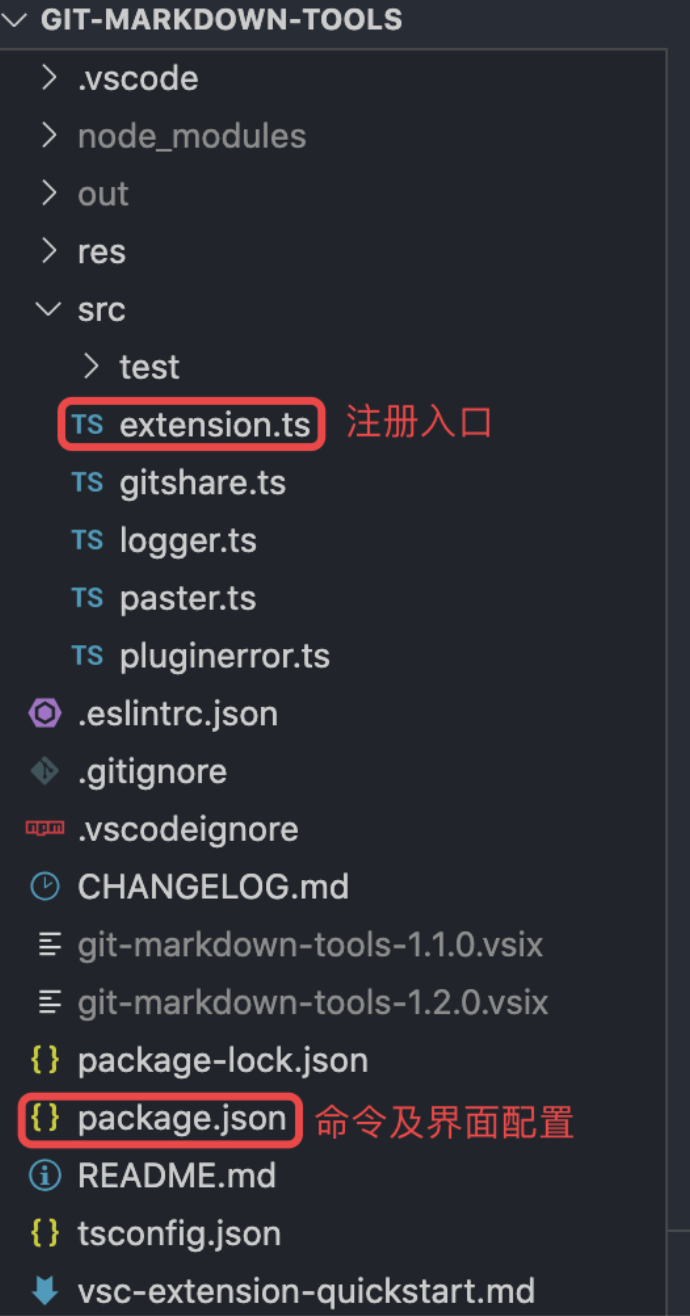
剪贴版复制图片,并设置默认大小
此功能是基于Paste Image插件的源码做的微调,新增img选择,如下所示:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21
| public static renderFilePath(languageId: string, basePath: string, imageFilePath: string): string { .... let imageSyntaxPrefix = ""; let imageSyntaxSuffix = ""; switch (languageId) { case "markdown": imageSyntaxPrefix = ``; break; case "asciidoc": imageSyntaxPrefix = `image::`; imageSyntaxSuffix = `[]`; break; case "img": imageSyntaxPrefix = `<img src="`; imageSyntaxSuffix = `" width = "500" />`; break; } .... return result; }
|
在右键菜单里添加相应的快捷入口,只需要配置package.json文件即可,如下所示:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33
| { .... "activationEvents": [ "onCommand:git-markdown-tools.MarkdownImagePastePrimary", "onCommand:git-markdown-tools.MarkdownImagePasteSubsidiary", ], "contributes": { "commands": [ { "command": "git-markdown-tools.MarkdownImagePastePrimary", "title": "粘贴图片-Primary", "category": "58IGit" }, { "command": "git-markdown-tools.MarkdownImagePasteSubsidiary", "title": "粘贴图片-Subsidiary", "category": "58IGit" } ], "menus": { "editor/context": [ { "command": "git-markdown-tools.MarkdownImagePastePrimary", "group": "navigation" }, { "command": "git-markdown-tools.MarkdownImagePasteSubsidiary", "group": "navigation" } ] } } }
|
Paste Image插件本身有一个问题:不支持直接复制本地的图片文件。
通过分析Paste Image的源码了解到,剪贴板的操作不是通过javascript实现的,而是通过调用对应平台的脚本实现:
- mac:AppleScript脚本
- linux:shell脚本
在macOS里,直接复制图片文件到剪贴板时,剪贴板里只有相应的文件路径,没有相应的图片内容,Paste Image的AppleScript脚本不支持这种类型,这个是后续需要修改点。
获取当前Markdown的远程Git地址
这个功能非常简单,主过程是如下:
- 先获取当前Markdown文件相对路径
- 拼接上相应服务器的地址即可
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
| public static copyRemoteUrlToClipboard(){ let editor = vscode.window.activeTextEditor; if(!editor) { return; }
let fileUri = editor.document.uri; if(!fileUri) { return; }
let filePath = fileUri.fsPath; let projectPath = vscode.workspace.rootPath; let fileRelativePath = filePath.replace(projectPath ?? '', '');
let projectRomoteUrlConfig = vscode.workspace.getConfiguration('git-markdown-tools')['romoteurl']; if (!projectRomoteUrlConfig) { Logger.showErrorMessage(`The config git-markdown-tools.romoteurl = '${projectRomoteUrlConfig}' is invalid. please check your config.`); return; }
let remoteUrl = GitShare.getRemoteUrl(projectRomoteUrlConfig, 'master', fileRelativePath);
vscode.env.openExternal(vscode.Uri.parse(remoteUrl)); }
private static getRemoteUrl(projectRomoteUrl: string, branch: string, fileRelativePath: string): string{ return `${projectRomoteUrl}/blob/${branch}${fileRelativePath}`; }
|
在右键菜单里添加相应的快捷入口,只需要配置package.json文件即可,如下所示:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30
| { ... "activationEvents": [ "onCommand:git-markdown-tools.CopyRemoteUrlToClipboard" ], "contributes": { "commands": [ { "command": "git-markdown-tools.CopyRemoteUrlToClipboard", "title": "打开远程Master地址", "category": "58IGit" } ], "menus": { "editor/title/context": [ { "command": "git-markdown-tools.CopyRemoteUrlToClipboard", "group": "navigation" } ], "editor/context": [ { "command": "git-markdown-tools.CopyRemoteUrlToClipboard", "group": "navigation" } ] } } }
|
GitHub开源地址
- 源码地址:https://github.com/handsomeliuyang/vscode-git-markdown-tools.git
- 插件安装地址:https://marketplace.visualstudio.com/items?itemName=handsomeliuyang.git-markdown-tools&ssr=false#overview
参考
- vscode-paste-image
- Your First Extension
- vscode-gitlens